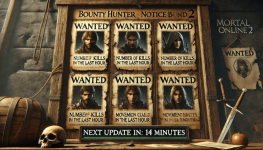
Proposed Improvement for the Bounty Hunter System in Mortal Online 2
Currently, many bounty hunters capture players who are AFK inside strongholds or houses, which devalues the experience and frustrates players looking for PvP. To address this issue and make the feature more engaging, I suggest an improved algorithm that will revolutionize how bounty hunters operate.
What's wrong today:
- Low mobility targets: Many targets are inactive in safe locations like houses or strongholds, making the hunt ineffective and frustrating for players seeking action.
- Repetitive targets: Hunting the same player multiple times or players with little activity decreases the excitement and challenge.
- Lack of meaningful incentives: The absence of significant rewards makes the bounty hunter mechanic lose appeal among the PvP community.
How the suggested changes improve the game:
- Dynamic and organic PvP: The improvement in the bounty hunter mechanic will attract top PvP players, who will now have clear incentives to hunt active player killers. This means less frustration for those looking for challenging fights and more real PvP in less time.
- Reduced griefing of new players: With top PvP players focused on hunting PKs, griefing of new players will decrease, as skilled fighters will be occupied with meaningful targets.
- Attracting new players: A well-implemented bounty hunter system, with intelligent target selection and meaningful rewards, will be a huge draw. Many players have a "bounty hunter" fantasy, inspired by western movies, and a game that executes this correctly will be irresistible to that audience.
New Bounty Hunter Algorithm
The algorithm will be optimized to find targets based on parameters that indicate activity, danger, and proximity. Each search will return 5 appropriate targets based on the following variables:- moving_last_hour: Distance (in meters) the red player moved in the last hour.
- inside_house_last_hour: Time (in minutes) the red player spent inside a house or stronghold in the last hour.
- kills_last_hour: Number of kills committed by the red player in the last hour.
- distance_km: Current distance (in kilometers) of the red player from the bounty hunter.
- possible_helpers_nearby: Number of allies, friends, or guild members near the red player within a 500-meter radius.
- killed_today: Number of times the red player has been killed today.
- killed_by_me_week: Number of times the bounty hunter has killed the red player this week.
- not_from_my_guild_and_aly: Boolean, true if the red player is not from the bounty hunter's guild or alliance.
Algorithm Logic:
- not_from_my_guild_and_aly must be true.
- killed_by_me_week, killed_today, and possible_helpers_nearby should be as low as possible to prioritize new and challenging targets.
- distance_km: The closer, the better to promote fast action.
- kills_last_hour and moving_last_hour: Targets that have caused more deaths and moved more will be prioritized to ensure active and dangerous targets.
- inside_house_last_hour: Targets that spent less time in safe locations will be prioritized, encouraging an active and dynamic hunt.
Suggested Code: C++ Function for Bounty Hunter Target Selection
Here’s the C++ function that receives five bounty hunter target suggestions based on the defined parameters:
C++:
#include <vector>
#include <algorithm>
// Define a struct to store the red player's data
struct RedPlayer {
std::string name;
std::string guild;
int moving_last_hour; // meters moved in the last hour
int inside_house_last_hour; // minutes inside a house or stronghold in the last hour
int kills_last_hour; // number of kills in the last hour
int distance_km; // distance in kilometers
int possible_helpers_nearby; // number of allies/friends within 500 meters
int killed_today; // number of times killed today
int killed_by_me_week; // number of times killed by the hunter this week
bool not_from_my_guild_and_aly; // true if not from my guild/alliance
};
// Function to compare two players based on our criteria
bool comparePlayers(const RedPlayer& a, const RedPlayer& b) {
// If player is not from my guild or alliance, prioritize
if (a.not_from_my_guild_and_aly != b.not_from_my_guild_and_aly) {
return a.not_from_my_guild_and_aly;
}
// Prioritize based on the number of times killed this week (lower is better)
if (a.killed_by_me_week != b.killed_by_me_week) {
return a.killed_by_me_week < b.killed_by_me_week;
}
// Prioritize based on the number of times killed today (lower is better)
if (a.killed_today != b.killed_today) {
return a.killed_today < b.killed_today;
}
// Prioritize based on the number of possible helpers nearby (lower is better)
if (a.possible_helpers_nearby != b.possible_helpers_nearby) {
return a.possible_helpers_nearby < b.possible_helpers_nearby;
}
// Prioritize based on distance (closer is better)
if (a.distance_km != b.distance_km) {
return a.distance_km < b.distance_km;
}
// Prioritize based on kills in the last hour (higher is better)
if (a.kills_last_hour != b.kills_last_hour) {
return a.kills_last_hour > b.kills_last_hour;
}
// Prioritize based on time spent inside a house (lower is better)
if (a.inside_house_last_hour != b.inside_house_last_hour) {
return a.inside_house_last_hour < b.inside_house_last_hour;
}
// Prioritize based on movement in the last hour (higher is better)
return a.moving_last_hour > b.moving_last_hour;
}
// Function to get the top 5 bounty targets
std::vector<RedPlayer> getTopBountyTargets(std::vector<RedPlayer>& redPlayers) {
// Sort the redPlayers based on our comparison criteria
std::sort(redPlayers.begin(), redPlayers.end(), comparePlayers);
// Return the top 5 results (or less if fewer players are available)
std::vector<RedPlayer> topTargets;
for (int i = 0; i < 5 && i < redPlayers.size(); ++i) {
topTargets.push_back(redPlayers[i]);
}
return topTargets;
}
Explanation of the C++ Function:
- Struct Definition: The RedPlayer struct stores all the necessary variables for each red player, including movement, kills, proximity to the hunter, and guild information.
- Comparison Logic: The comparePlayers function defines how to prioritize the bounty targets. It uses the criteria outlined (guild, kills, distance, etc.) to compare two players.
- Target Selection: The getTopBountyTargets function sorts the list of potential targets and returns the top 5, prioritizing based on the defined rules.
Other Suggested Improvements:
- Reduce the number of "Murder Counts" to 3: This will encourage more players to turn red and seek PvP responsibly, knowing they can avoid murder counts by using the /duel command for consensual fights.
- New Rewards: Bounty coins should offer meaningful rewards such as:
- 10 ravens
- 100 bandages
- 200 arrows
- Potions with 40 units (with 3dh)
- Kits with reagents for ecumenical and elementalism
- Other items like Cuprum Rechard and community suggestions.
- New Titles and Capes: Add personalized titles and capes, like a white cape or cloak for players who complete over a thousand bounty hunts. This will give long-term recognition to those who consistently participate in the system.
These changes will transform the Bounty Hunter system in Mortal Online 2 into one of the most dynamic and attractive features, promoting quality PvP, significant rewards, and creating an engaging gameplay cycle for the community.
Last edited: